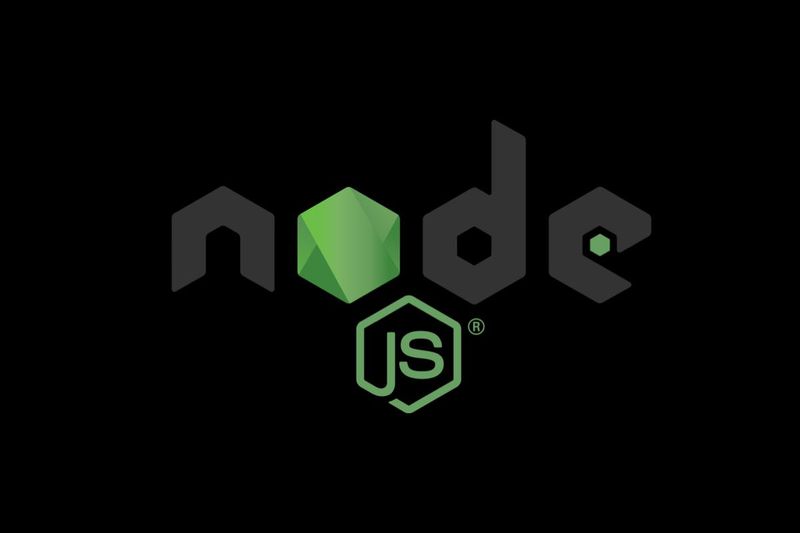
Node.js is a powerful JavaScript runtime that allows developers to work with files, directories, and asynchronous programming efficiently. This blog post explores how to read and write files, work with directories, and understand asynchronous programming concepts such as callbacks, Promises, and async/await.
1. Working with the File System in Node.js
The Node.js fs
(File System) module provides methods to interact with the file system, such as reading, writing, and managing files and directories.
1.1 Reading Files
To read a file in Node.js, you can use fs.readFileSync()
(synchronous) or fs.readFile()
(asynchronous).
Synchronous Reading:
const fs = require('fs'); const data = fs.readFileSync('example.txt', 'utf8'); console.log(data);
Asynchronous Reading:
fs.readFile('example.txt', 'utf8', (err, data) => { if (err) { console.error(err); return; } console.log(data); });
1.2 Writing Files
Similar to reading, you can write files synchronously or asynchronously.
Synchronous Writing:
fs.writeFileSync('output.txt', 'Hello, Node.js!'); console.log('File written successfully');
Asynchronous Writing:
fs.writeFile('output.txt', 'Hello, Node.js!', (err) => { if (err) { console.error(err); return; } console.log('File written successfully'); });
2. Working with Directories
You can create, read, and delete directories using the fs
module.
2.1 Creating a Directory
fs.mkdir('new_directory', (err) => { if (err) { console.error(err); return; } console.log('Directory created'); });
2.2 Reading a Directory
fs.readdir('.', (err, files) => { if (err) { console.error(err); return; } console.log('Files in directory:', files); });
2.3 Removing a Directory
fs.rmdir('new_directory', (err) => { if (err) { console.error(err); return; } console.log('Directory removed'); });
3. Introduction to Asynchronous Programming
JavaScript and Node.js rely heavily on asynchronous programming to handle operations like reading files, making API requests, and handling databases efficiently.
3.1 Callbacks
A callback is a function passed as an argument to another function and executed later.
Example:
function greet(name, callback) { console.log('Hello, ' + name); callback(); } greet('Obafemi', () => { console.log('This is a callback function'); });
3.2 Promises
A Promise represents a value that may be available now, later, or never. It has three states: pending, resolved, and rejected.
Example:
const fetchData = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve('Data fetched successfully'); }, 2000); }); }; fetchData().then((data) => console.log(data)).catch((err) => console.error(err));
3.3 Async/Await
async
and await
provide a more readable way to work with asynchronous code.
Example:
const fetchData = async () => { try { const data = await new Promise((resolve) => { setTimeout(() => resolve('Data fetched successfully'), 2000); }); console.log(data); } catch (error) { console.error(error); } }; fetchData();
Conclusion
In this blog post, we covered:
- How to read and write files in Node.js.
- How to work with directories.
- Basics of asynchronous programming, including callbacks, Promises, and async/await.
By understanding these concepts, you can write efficient and scalable applications in Node.js. Keep practicing, and happy coding!
Leave a Comment